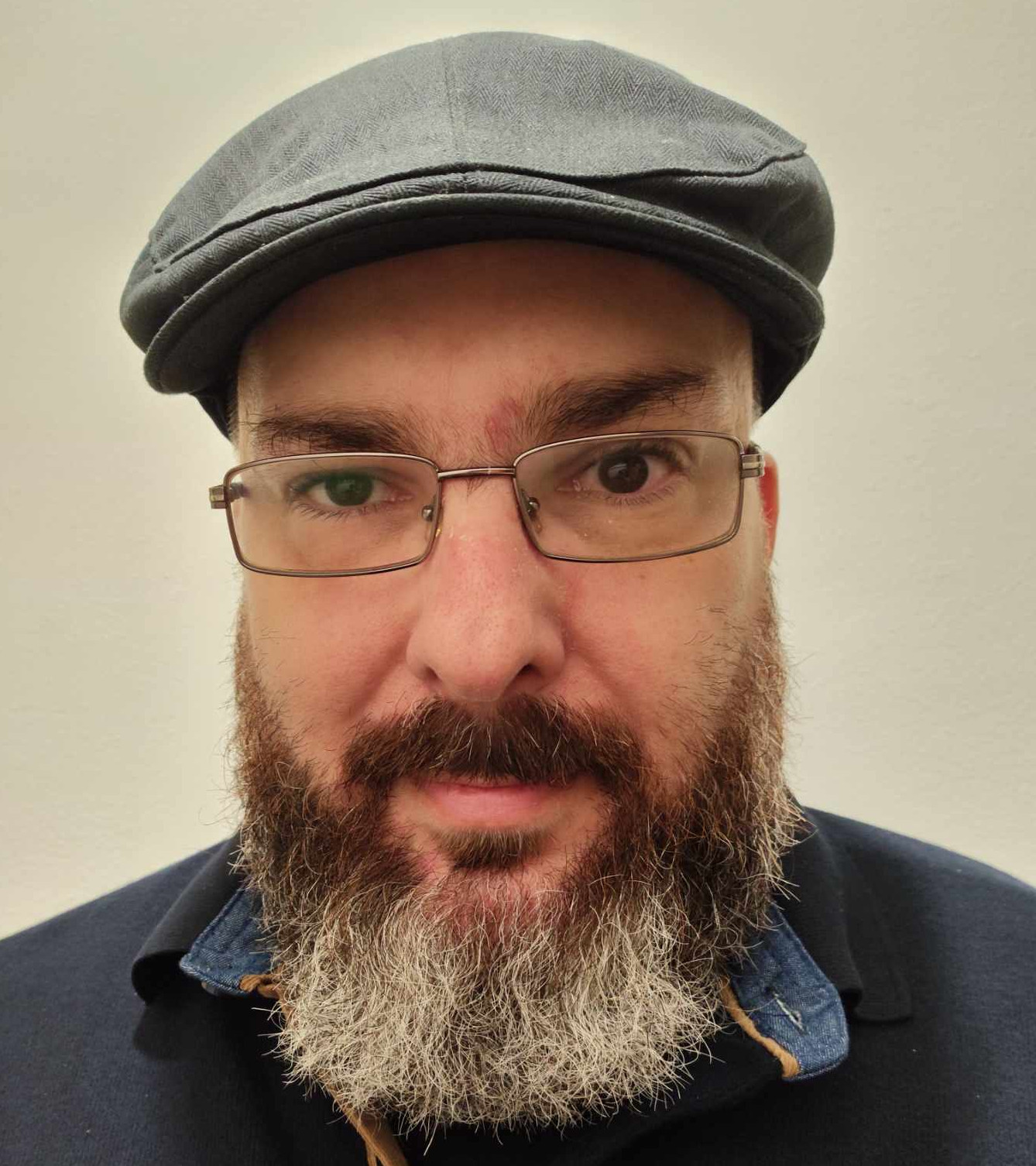
Thodoris Kouleris
Software Engineer
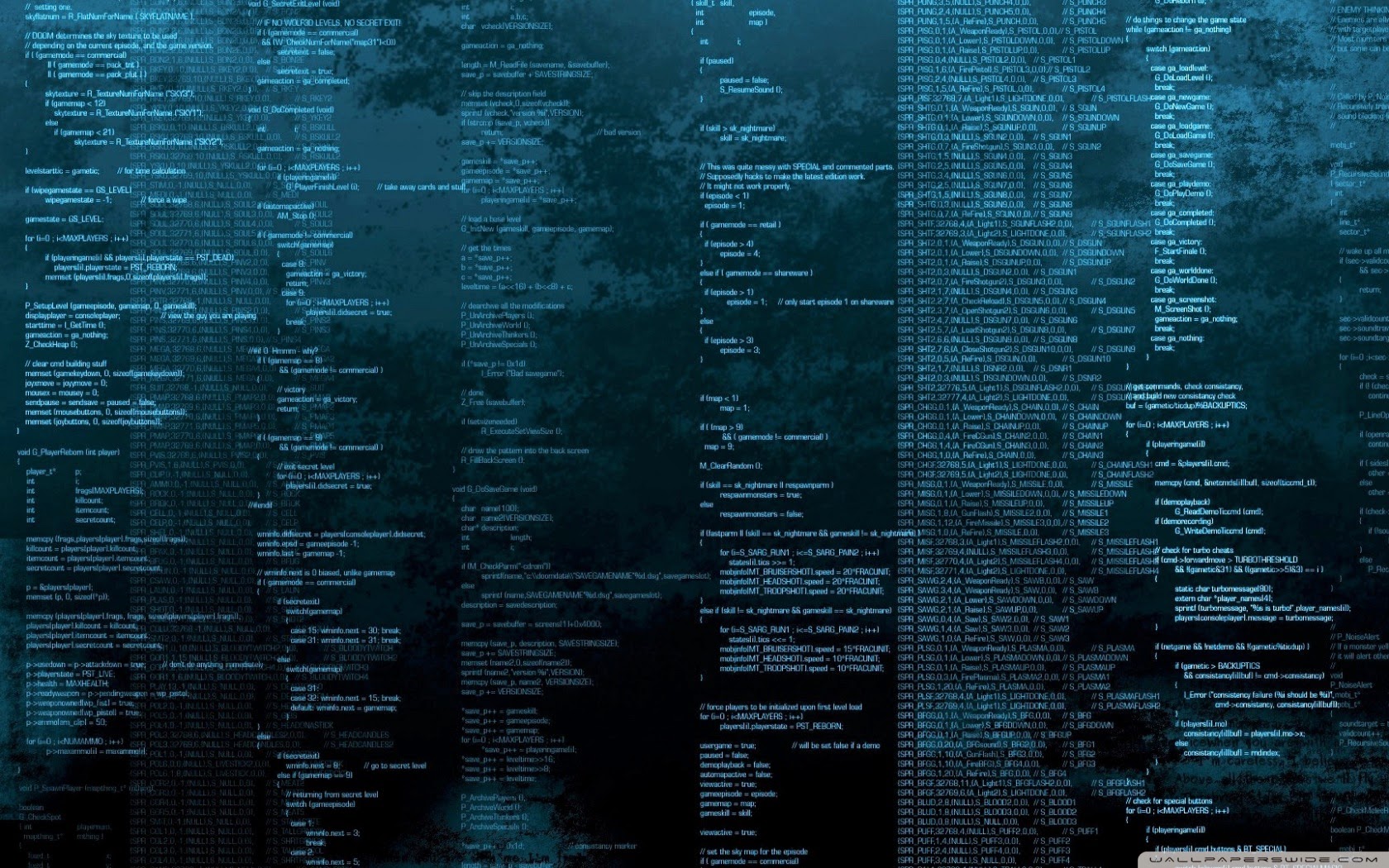
Sorting Algorithms #1: Bubble Sort
What are Sorting Algorithms?
Sorting algorithms are a set of algorithms that help us arrange data in ascending or descending order. They are fundamental to computer science, and the differences between them mainly lie in their time complexity and space complexity, which determine their suitability for handling larger datasets.
Bubble Sort
The simplest and, at the same time, the worst sorting algorithm is Bubble Sort. In Bubble Sort, we start from the beginning of the dataset and compare the first two elements. The smaller one is placed first, and the larger one second. Then, we compare the next two elements and repeat the process. This continues until all elements are compared. Once a full pass is complete, the process is repeated from the start until the dataset is fully sorted.
For example, consider the array [10, 4, 5, 8, 0]. To execute the algorithm: Compare 10 with 4. Since 4 is smaller, the array becomes [4, 10, 5, 8, 0]. Compare 10 with 5. Since 5 is smaller, the array becomes [4, 5, 10, 8, 0]. Compare 10 with 8. Since 8 is smaller, the array becomes [4, 5, 8, 10, 0]. Compare 10 with 0. Since 0 is smaller, the array becomes [4, 5, 8, 0, 10]. This completes the first pass. The process is repeated until the array is fully sorted.
Code
def bubble_sort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already sorted, no need to check them
for j in range(0, n-i-1):
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
data = [10, 4, 5, 8, 0]
sorted_data = bubble_sort(data)
print("Sorted array:", sorted_data)
Algorithm Complexity
The time complexity of Bubble Sort is O(n2) in the worst case, where n is the number of elements. This makes the algorithm unsuitable for large datasets.