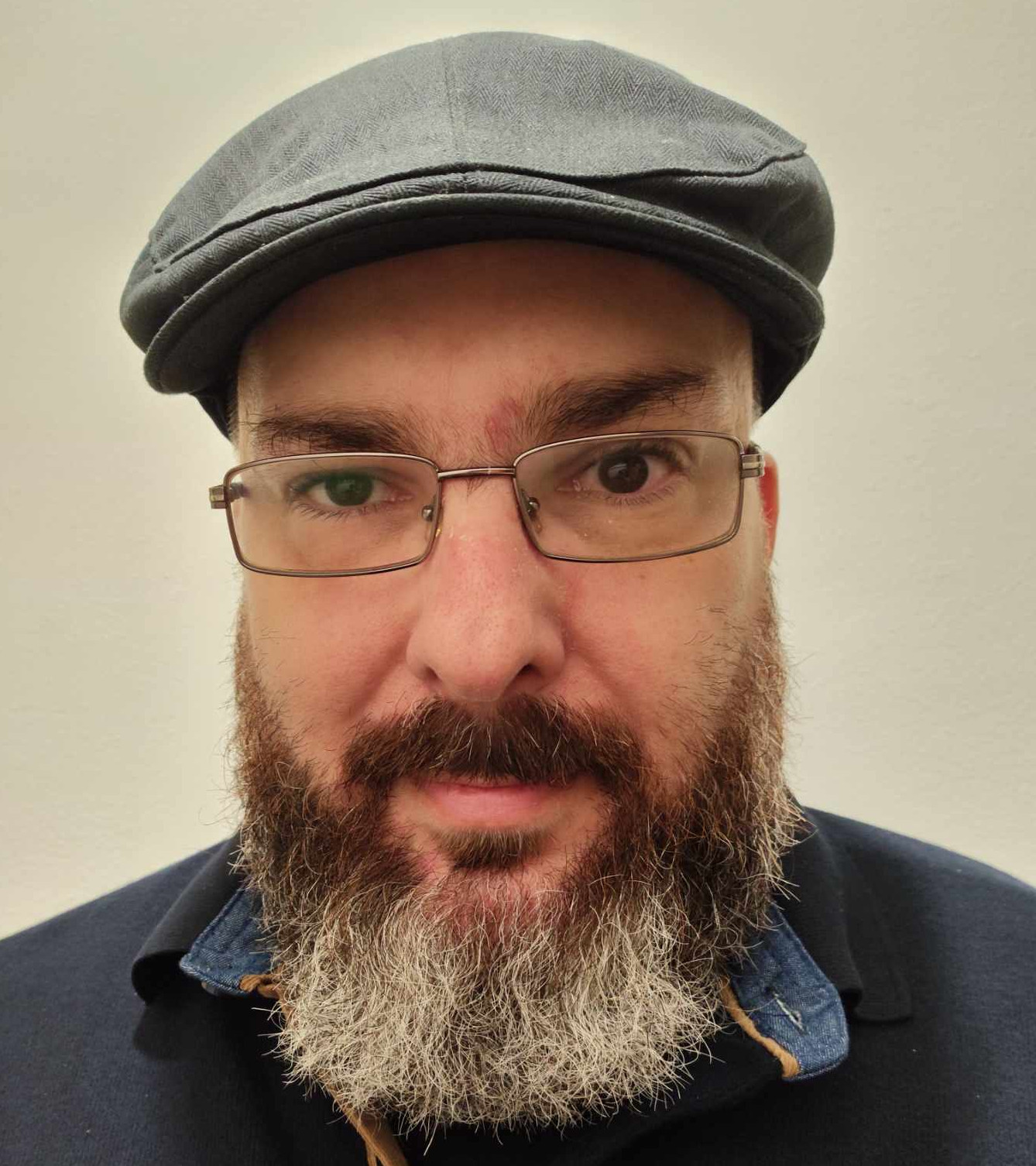
Thodoris Kouleris
Software Engineer
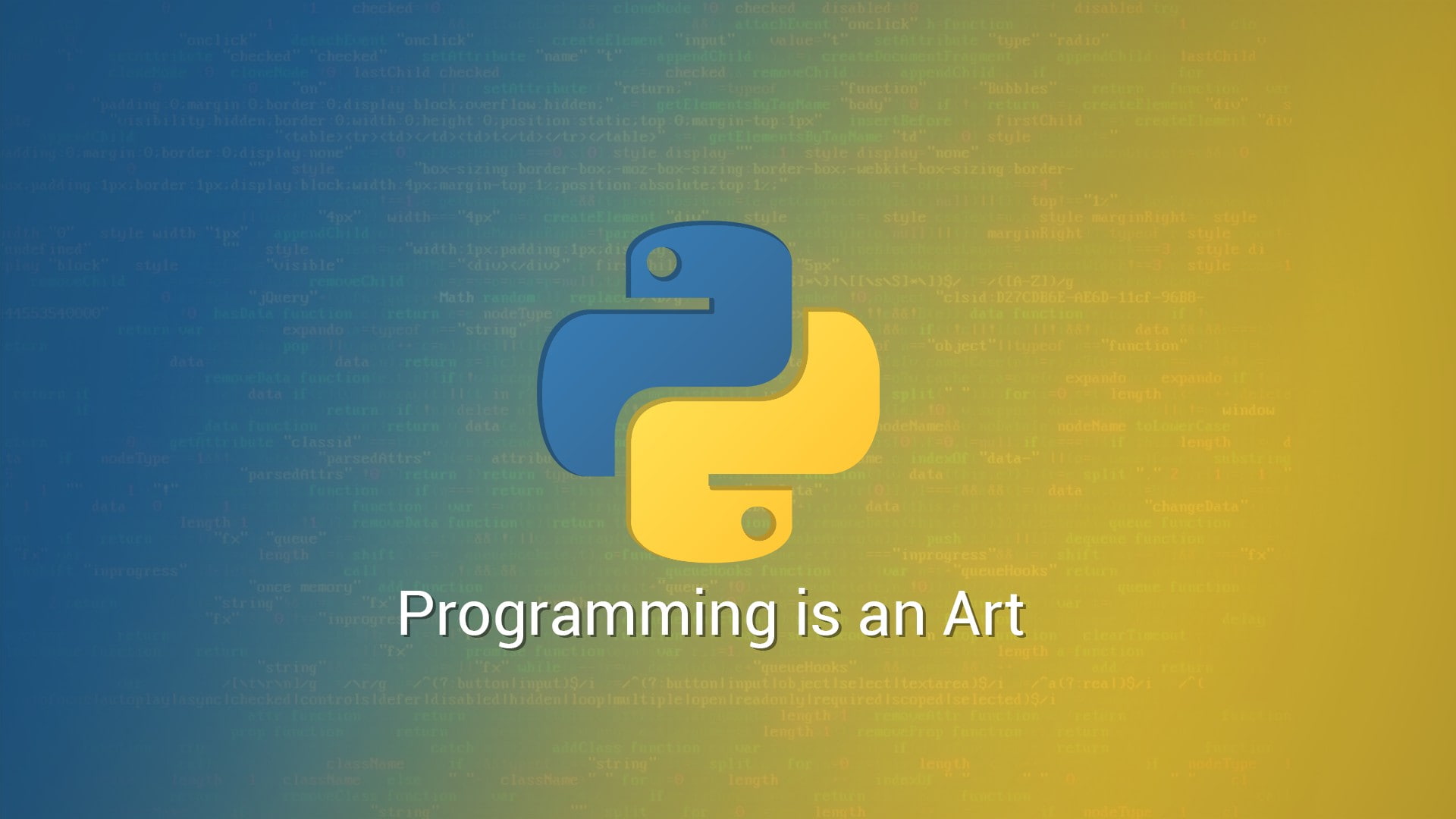
Python Tutorial #3: Lists, Tuples and sets
Lists
A list in Python is a data structure used to store collections of elements in a specific order. The elements in a list can be of any type, including numbers, strings, other lists, and other objects. A list is mutable, which means its elements can be modified after creation.
my_list = [1, 2, 3, 4, 5]
another_list = ["apple", "banana", "cherry"]
mixed_list = [1, apple, True, 3.14]
-
You can access list elements using indexes, starting from
0
for the first element.print(my_list[0]) # prints: 1 print(another_list[2]) # prints: cherry
-
You can modify list elements by assigning a new value to a specific index.
my_list[1] = 10 print(my_list) # prints [1, 10, 3, 4, 5]
-
You can add elements to a list using methods like
append()
to add an element at the end orinsert()
to add an element at a specific position.my_list.append(6) print(my_list) # prints [1, 10, 3, 4, 5, 6] my_list.insert(2, "new element") print(my_list) # prints [1, 10, 'new element', 3, 4, 5, 6 ]
-
You can remove elements from a list using the
remove()
method (to remove a specific element) or thepop()
method (to remove an element by index or the last element).my_list.remove(10) print(my_list) # prints [1, 'new element', 3, 4, 5, 6] my_list.pop(2) print(my_list) # prints [1, 'new element', 4, 5, 6]
Tuples
A tuple in Python is a data structure similar to a list but with one key difference: tuple elements are immutable. This means that once a tuple is created, you cannot change, add, or remove elements from it. Tuples are used when you want to group data and ensure that it cannot be modified.
my_tuple = (1, 2, 3)
another_tuple = ("apple", "banana", "cherry")
mixed_tuple = (1, "apple", True, 3.14)
-
Like lists, you can access tuple elements using indexes.
print(my_tuple[0]) # prints 1 print(another_tuple[2]) # prints cherry
-
Tuple elements cannot be modified after creation. Any attempt to do so will result in an error.
my_tuple[1] = 10 # it will cause a TypeError
Sets
A set in Python is a collection of unique elements without a specific order. Unlike lists and tuples, sets do not allow duplicate elements, and their elements have no defined order. These characteristics make sets ideal for situations where you need to store unique values and perform fast operations like unions, intersections, and differences.
my_set = {1, 2, 3, 4}
another_set = {"apple", "banana", cherry"}
empty_set = set()
-
You can add elements to a set using the
add()
method.my_set.add(5) print(my_set) # prints {1, 2, 3, 4, 5}
-
You can remove elements using the
remove()
ordiscard()
methods. Theremove()
method will raise an error if the element does not exist, whilediscard()
will not.my_set.remove(3) print(my_set) # prints {1, 2, 4, 5}