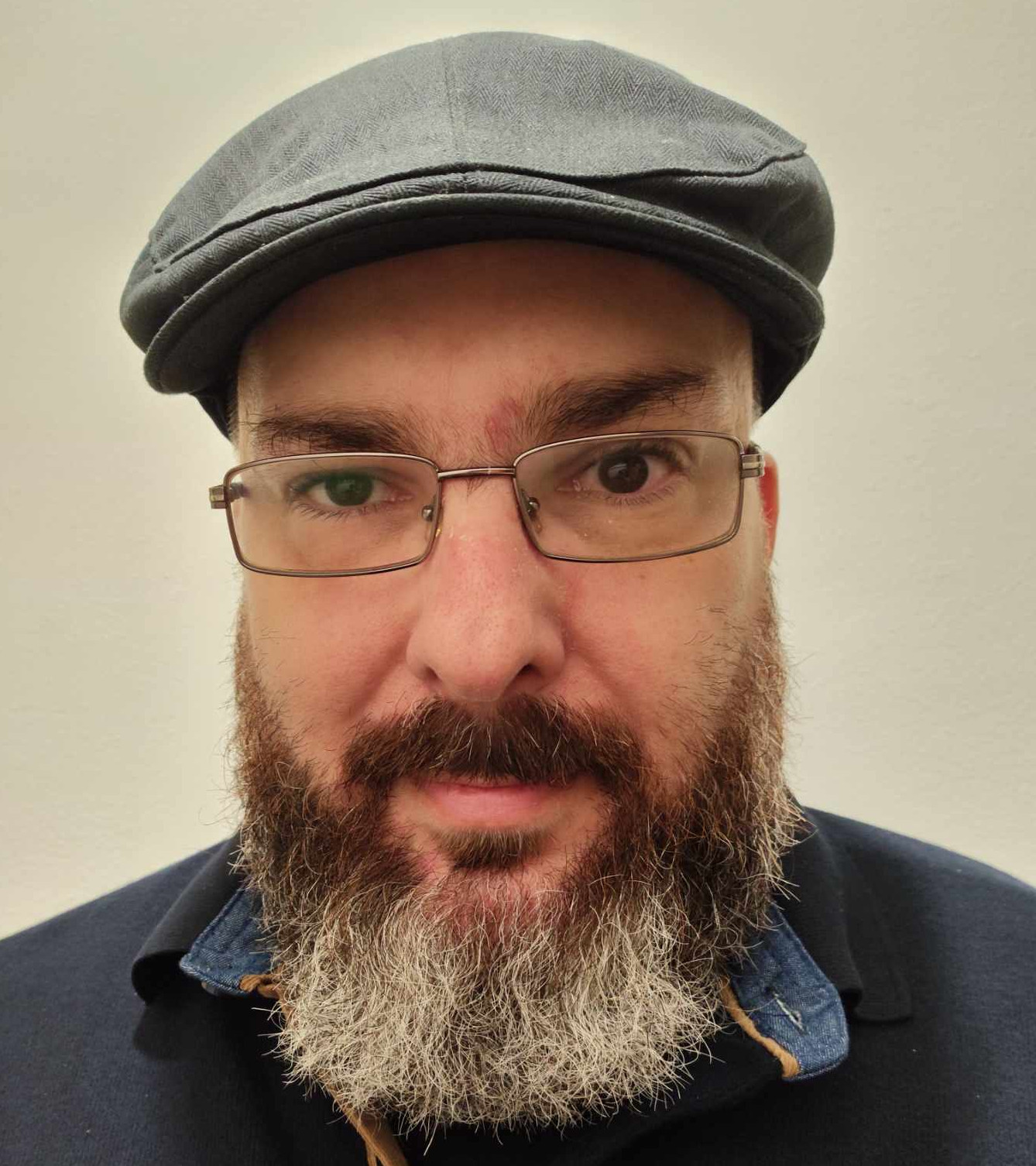
Thodoris Kouleris
Software Engineer
© 2024 Thodoris Kouleris
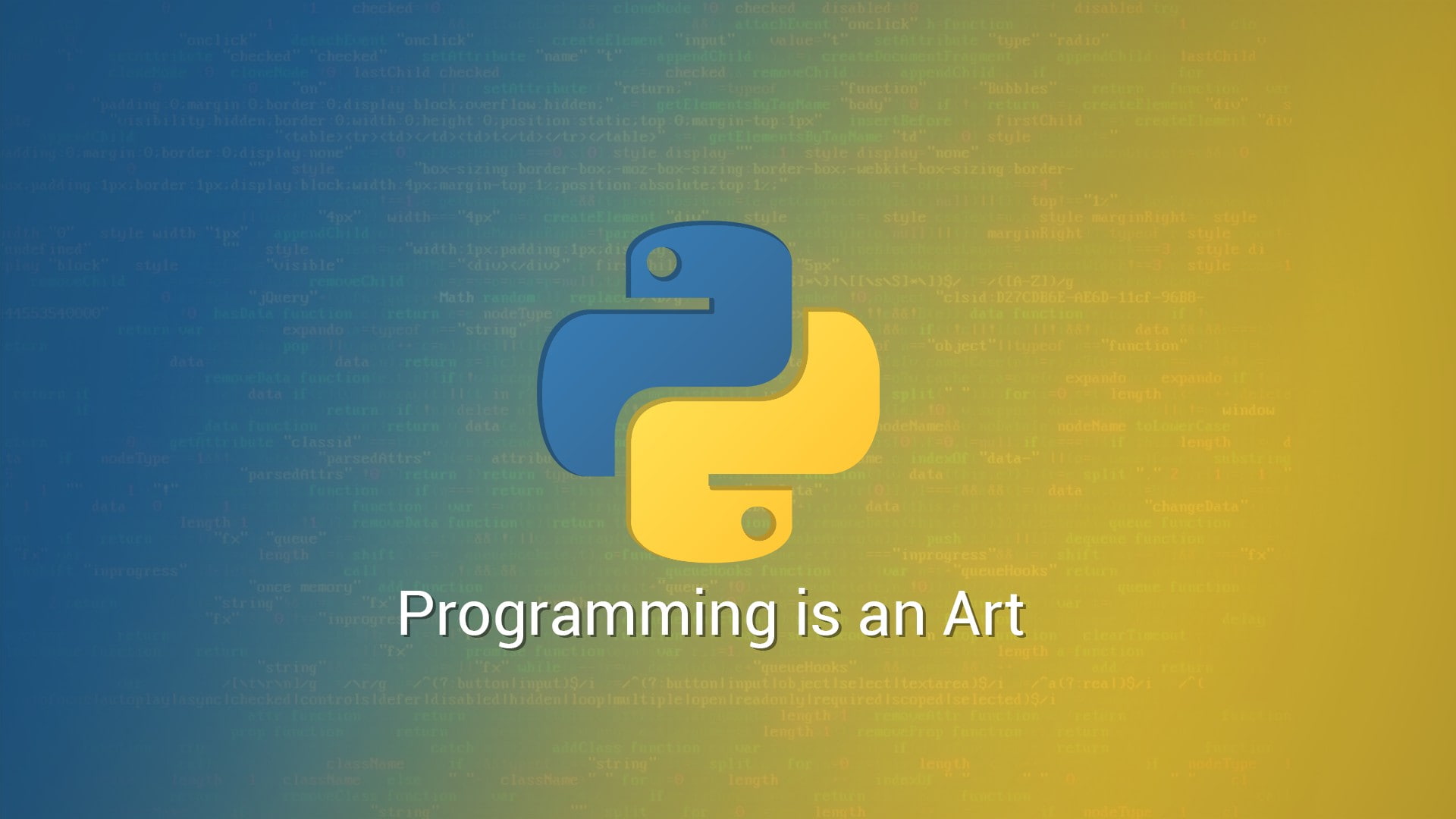
Python Tutorial #4: Booleans
Boolean values in Python are a data type that can only take two values: True
or False
.
These values are often used to control conditions in logical expressions, loops, and control structures such as
if
, while
, and for
statements.
Characteristics of Booleans in Python
- The type of Boolean values is
bool
. - In Python, comparisons, logical operations, and many other functions return Boolean values.
- Everything can be evaluated as
True
orFalse
: Numbers:0
is consideredFalse
, while any other number isTrue
. Lists, strings, and other containers: Empty ones areFalse
, while non-empty ones areTrue
.
# boolean values
x = True
y = False
print( 5 > 3 ) # True
print(2 == 5 ) # False
if x:
print("x value is True")
else:
print("x value is False")
Boolean values are fundamental for controlling the flow of programs.