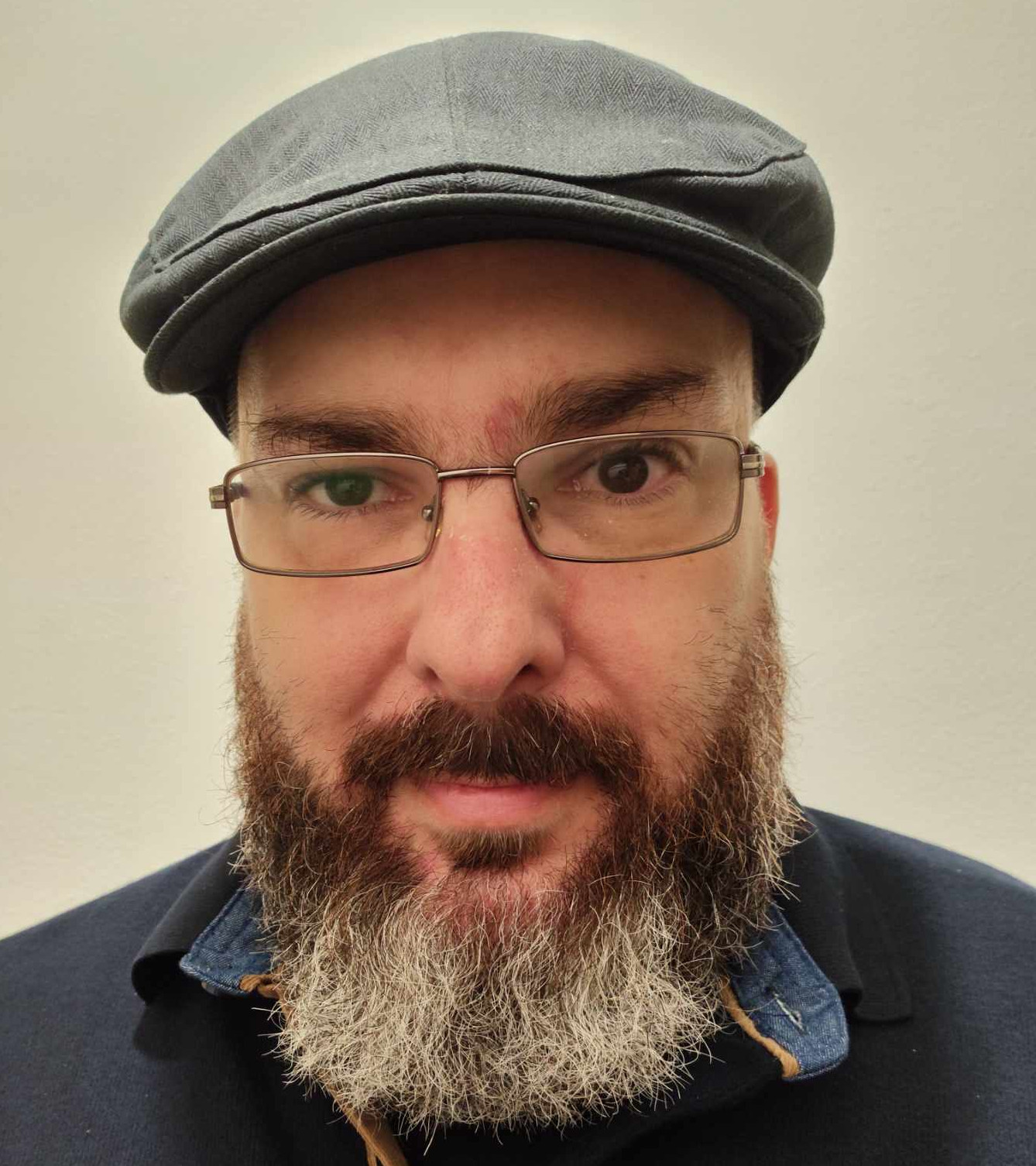
Thodoris Kouleris
Software Engineer
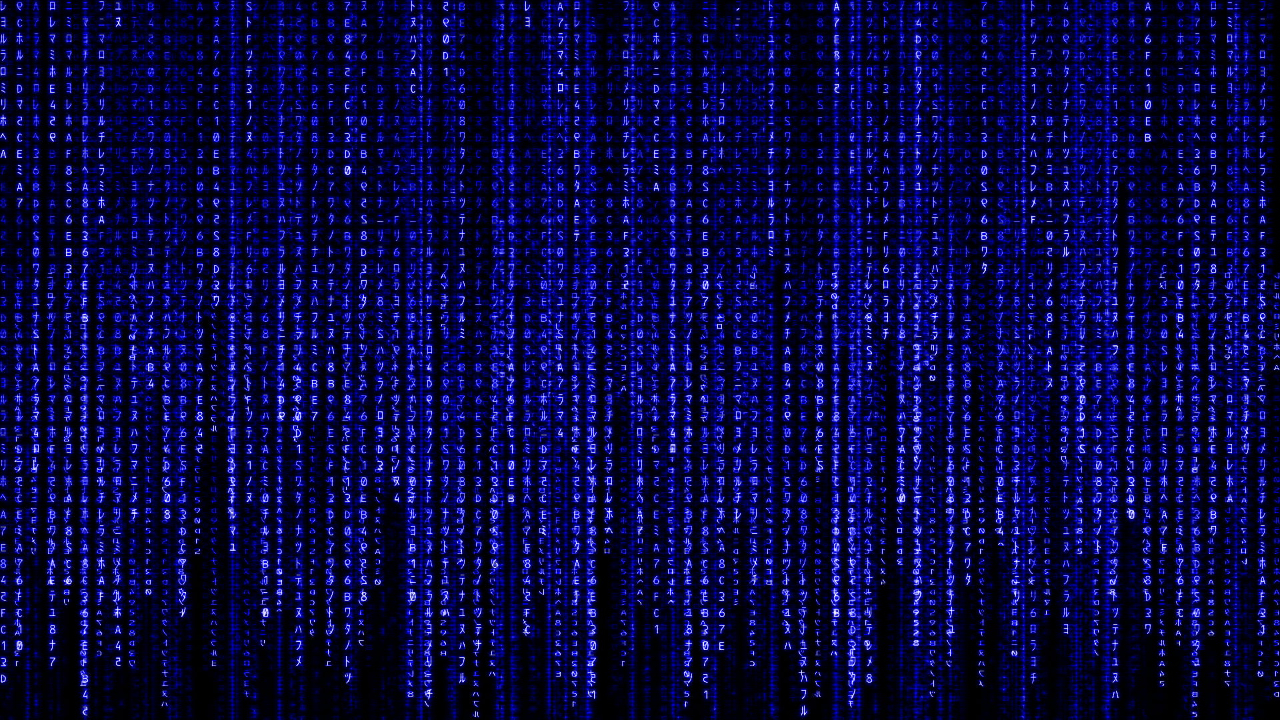
Singleton Pattern
The Singleton Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance.
There will be times that as a programmer you will only need one object of a specific class in order either to control access to a resource like database connection, logging service or configuration manager or to one global object for the entire application. Without the singleton pattern, you risk creating multiple instances of a class that should really only exist once.
How it works
The simplest way to restrict the creation of multiple objects is to restrict the source of the object creation of a class aka the constructor. Your class should have an private class instance property and you constructor should also be private. You must have a method of getInstance that will return the instance property. When someone calls the getInstance the method will check if the instance property is null and if it is null will create an instance of the class. If the instance property is not null there it returns it.
Python Example
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # True
Java Example
public class Singleton {
private static Singleton instance;
// Private constructor prevents instantiation from other classes
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Problems
The most significant problem with the singleton pattern is that you have a global state and it makes the unit testing difficult. It also makes it easier to violate the single responsibility principle.