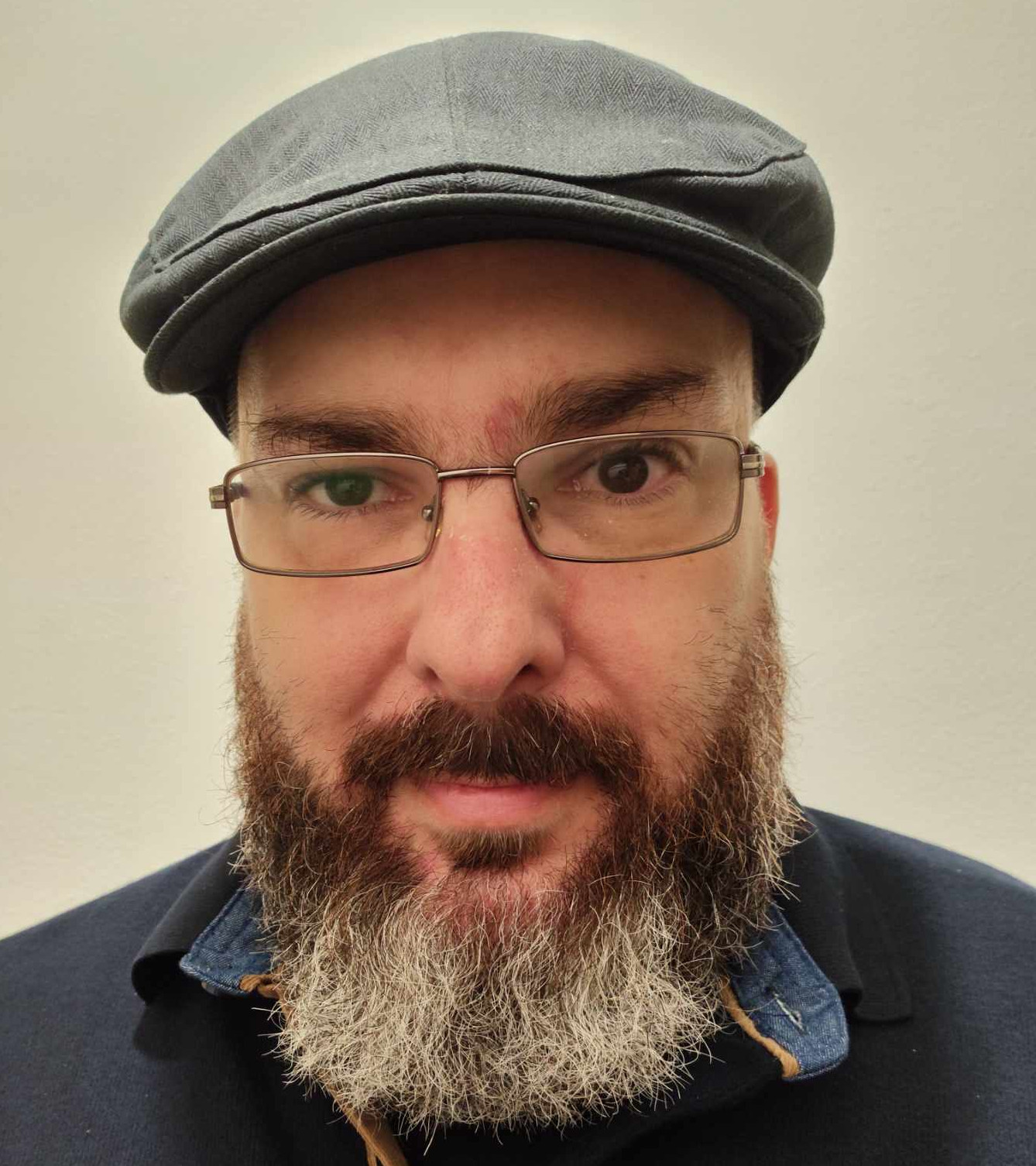
Thodoris Kouleris
Software Engineer
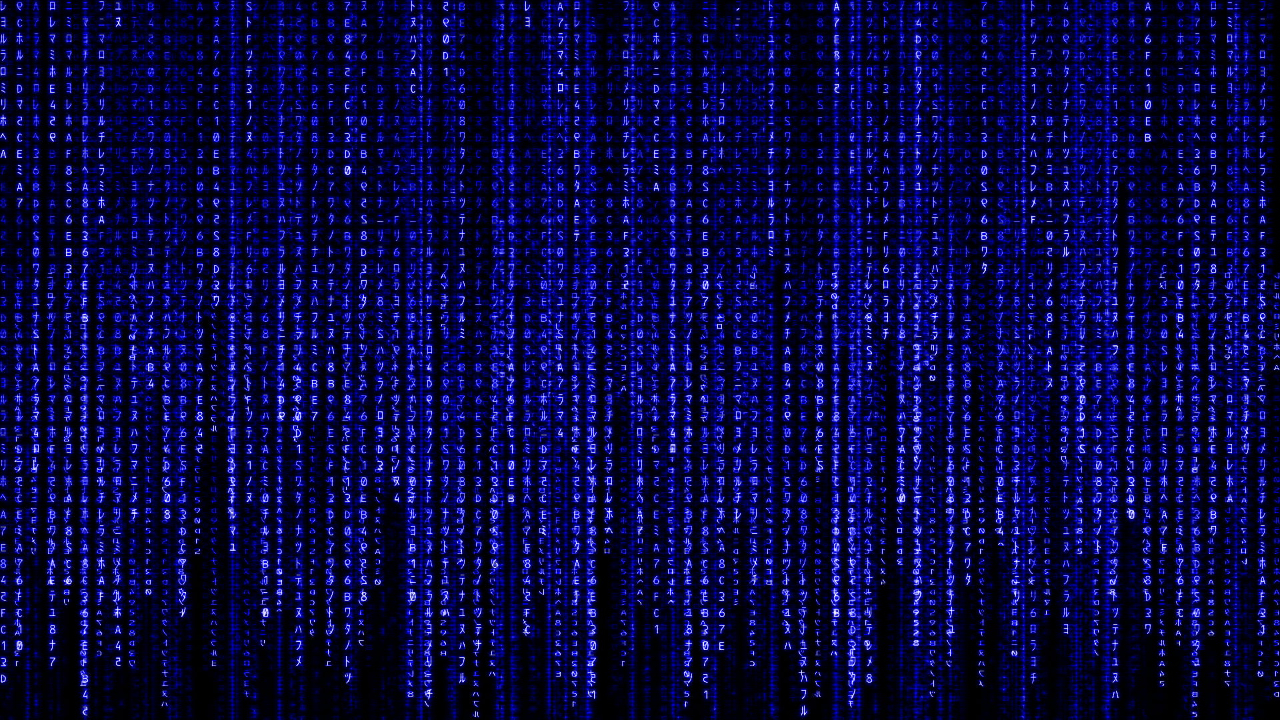
The Repository Pattern (Java / PHP)
The Repository Pattern is a design pattern commonly used in software development, particularly in the field of data management. Its purpose is to provide an additional abstraction layer for accessing data, ensuring that the code handling application logic remains independent of the storage system implementation.
Repository Pattern in Java
In Java, the Repository Pattern can be used to manage interactions with the database. Here’s an example with a UserRepository
:
public interface UserRepository {
User findById(int id);
List findAll();
void save(User user);
void delete(int id);
}
public class JdbcUserRepository implements UserRepository {
// JDBC implementation
}
public class HibernateUserRepository implements UserRepository {
// Hibernate implementation
}
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(int id) {
return userRepository.findById(id);
}
}
Here, the UserService
depends on UserRepository
but does not know the actual implementation. This allows for easy replacement with different implementations (e.g., JdbcUserRepository
, HibernateUserRepository
).
Repository Pattern in PHP
In PHP, a similar structure can be used. Here’s an example with a UserRepository
:
interface UserRepository {
public function findById(int $id): User;
public function findAll(): array;
public function save(User $user): void;
public function delete(int $id): void;
}
class MySqlUserRepository implements UserRepository {
// MySQL-specific implementation
}
class UserService {
private UserRepository $userRepository;
public function __construct(UserRepository $userRepository) {
this->userRepository = $userRepository;
}
public function getUserById(int $id): User {
return $this->userRepository->findById($id);
}
}
Here too, UserService
uses UserRepository
without concerning itself with the actual implementation. This allows flexibility in replacing MySqlUserRepository
with other implementations.
Summary
The Repository Pattern provides efficient data and storage management while promoting flexibility and maintainability in software development, whether in Java or PHP.